๐ 101-Mastering Python Sets: The Unordered Heroes of Data Storage! ๐
PYTHON
9/1/20243 min read
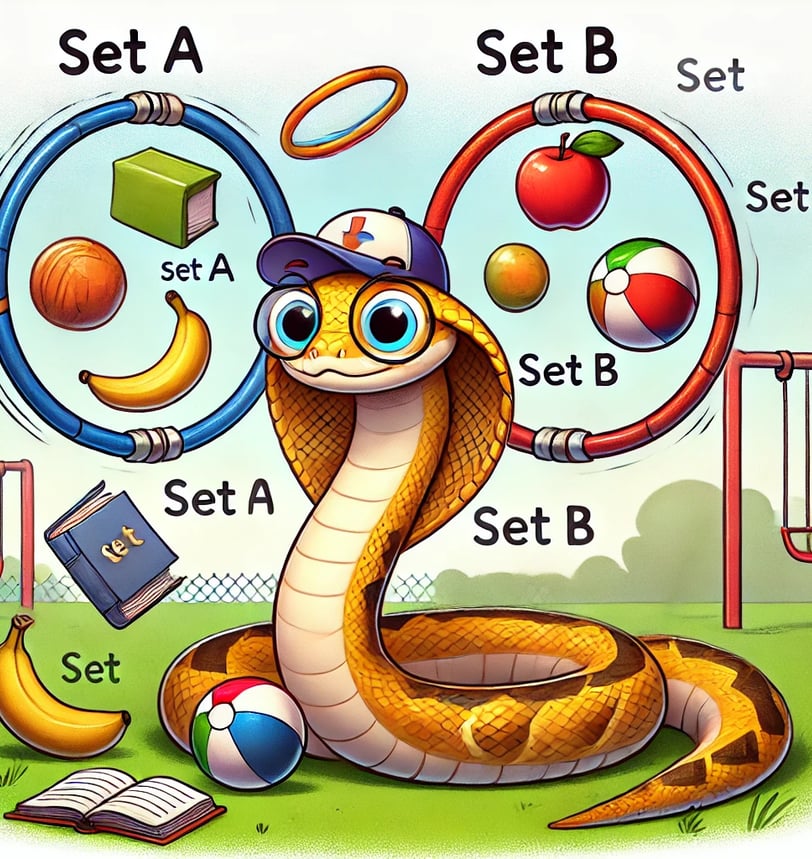
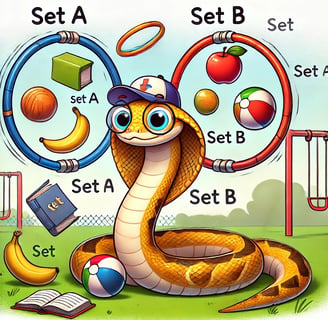
Hello, Python learners! ๐ Today, we are diving into the world of sets in Python. Sets are a unique and powerful data structure that offers some fantastic features for managing collections of data. So, let's learn everything about sets with easy-to-understand explanations and fun examples! Ready? Letโs go! ๐
What is a Set in Python? ๐ค
A set is a collection in Python that is:
Unordered: The elements do not have a defined order, which means you cannot access items using an index.
Unchangeable (immutable elements): While the set itself is mutable (you can add or remove elements), the elements contained in a set must be immutable (like strings, numbers, or tuples).
Unique: All elements in a set are unique; duplicates are automatically removed.
Analogy:
Think of a set like a bag of mixed candies ๐๐ฌ. You can shake the bag, and it doesnโt matter how theyโre arranged; you only care about what types of candies are inside and that you don't have any duplicates!
Creating a Set ๐ ๏ธ
There are two main ways to create a set in Python: using curly braces {} or the set() function.
Method 1: Using Curly Braces
Method 2: Using the set() Function
Note: The set() function removes duplicates from the input iterable, hence why {1, 2, 2, 3, 4} becomes {1, 2, 3, 4}.
Accessing Set Elements ๐
Because sets are unordered, you cannot access elements by their position (like you can with lists or tuples). However, you can check if an element exists in a set using the in keyword:
Adding and Removing Elements โ
Adding Elements
You can add elements to a set using the add() method:
Removing Elements
To remove elements, you can use the remove(), discard(), or pop() methods:
Tip: Use discard() instead of remove() if you want to avoid potential KeyError exceptions when an element is not found.
Set Operations ๐งฎ
Sets in Python support mathematical operations like union, intersection, difference, and symmetric difference.
Union (| or union())
Combines all unique elements from both sets:
Intersection (& or intersection())
Gets only the elements that are in both sets:
Difference (- or difference())
Finds elements that are in the first set but not in the second:
Symmetric Difference (^ or symmetric_difference())
Finds elements that are in either of the sets, but not in both:
Set Methods ๐ ๏ธ
Python provides several built-in methods to work with sets:
add(): Adds an element to the set.
remove(): Removes a specific element; raises KeyError if not found.
discard(): Removes a specific element; does nothing if not found.
pop(): Removes and returns an arbitrary element.
clear(): Removes all elements from the set.
union(): Returns the union of two sets.
intersection(): Returns the intersection of two sets.
difference(): Returns the difference of two sets.
symmetric_difference(): Returns the symmetric difference of two sets.
Example of Set Methods
Looping Through a Set ๐
You can loop through a set to access its elements:
Checking Membership ๐
To check if an element exists in a set, use the in keyword:
Real-World Use Cases ๐
Sets are useful in a variety of scenarios:
1. Removing Duplicates from a List ๐ฏ
Sets are great for removing duplicates because they automatically discard duplicate values:
3. Mathematical Operations ๐งฎ
Sets are often used to perform common mathematical operations, like finding the union or intersection of datasets.
Summary ๐
Sets are unordered collections of unique elements.
Sets are mutable; you can add or remove elements.
Sets are ideal for removing duplicates and performing mathematical set operations like union, intersection, difference, and symmetric difference.
Python provides several methods to efficiently work with sets.
2. Membership Tests ๐ฆ
Sets are optimized for fast membership tests, making them ideal for situations where you need to frequently check the presence of an item:
๐ง Test Your Knowledge with Kahoot!
Thank you for learning about Python with us! To make this learning experience even more interactive, we invite you to test your Python knowledge by playing our Kahoot quiz. Click the link below to get started and challenge yourself!
๐ฎ Play the Python Intro Quiz on Kahoot!
Have fun and see how much you've learned! ๐