π 101-Python Conditions: Mastering if, elif, and else! π
PYTHON
8/30/20242 min read
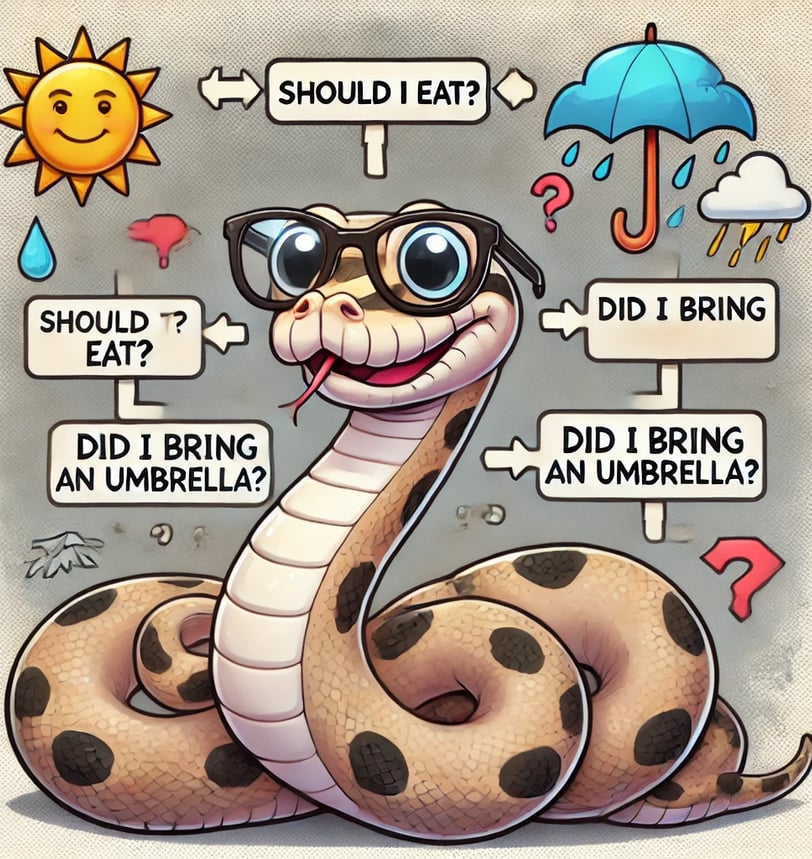
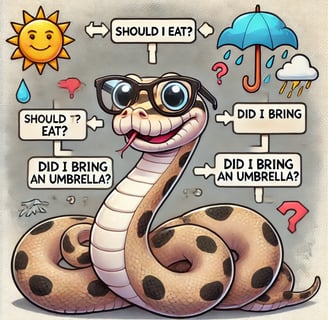
Hello, Pythonistas! π Today, we're diving into the world of conditional statements in Python: if, elif, and else. These powerful tools let us make decisions in our code, guiding the flow based on different conditions. Think of them like crossroads β depending on the condition, you choose which path to follow!
π§ Why Use Conditional Statements?
Conditional statements help you control the flow of your program. They allow you to execute certain code blocks only when specific conditions are met. This is super useful when you want your program to react differently based on user input or other variables!
π The Basics: if Statement
The if statement is like asking a question: "If this condition is true, do something." Let's see how it works:
In this example, the condition age >= 18 checks if the age is 18 or older. If it is, the program prints "You are eligible to vote!"
π Adding More Conditions: elif (else if)
What if we want to check more than one condition? Thatβs where elif comes in! It allows us to check another condition if the first one isn't true.
Here, if the first if condition (age >= 18) is false, the program checks the elif condition (age == 16 or age == 17). If this condition is true, it prints "You are almost old enough to vote!"
π¨ The Default Action: else
What if none of the conditions are met? That's when else comes into play. Itβs like a catch-all for anything that doesnβt match the previous conditions.
If age is 15, both if and elif conditions are false, so the program executes the else block and prints "You are not old enough to vote."
π― Combining Conditions with Logical Operators
You can combine multiple conditions using logical operators like and, or, and not.
Here, both conditions (is_raining and not have_umbrella) must be true for the message to print.
π Nested if Statements
You can also place an if statement inside another if statement. This is called nesting.
In this nested example, the program first checks if age >= 18. If true, it checks if has_id is also true. If both conditions are met, it prints "You can enter the club!"
π Using if, elif, and else in Real-World Applications
Let's explore some real-world scenarios where you might use these conditionals:
Example 1: Determining the Grade of a Student
This program determines the grade based on the student's score. If the score is 85, the output will be "Grade: B".
Example 2: Checking Stock Levels
Here, the program checks the stock level and prints a message accordingly. With stock = 5, the output is "Stock level is low."
Example 3: User Login System
This simple login system checks if the input username and password match the stored ones. If they do, it prints "Login successful!".
π οΈ Practice Time!
Try modifying the examples above to test your understanding! Can you create a program that checks multiple conditions? What about one that uses both elif and nested if statements?
π Ready to Test Your Knowledge?
Put your newfound skills to the test with a fun quiz! Check out this Kahoot Quiz to see how well you understand Python conditional statements!
π’ Share Your Progress!
Share this post with your friends and challenge them to master Python conditionals too! π²π»
Facebook: Share on Facebook
LinkedIn: Share on LinkedIn
Happy coding! π