๐ 101-Python Functions: A Comprehensive Guide with Fun Examples
PYTHON
8/30/20242 min read
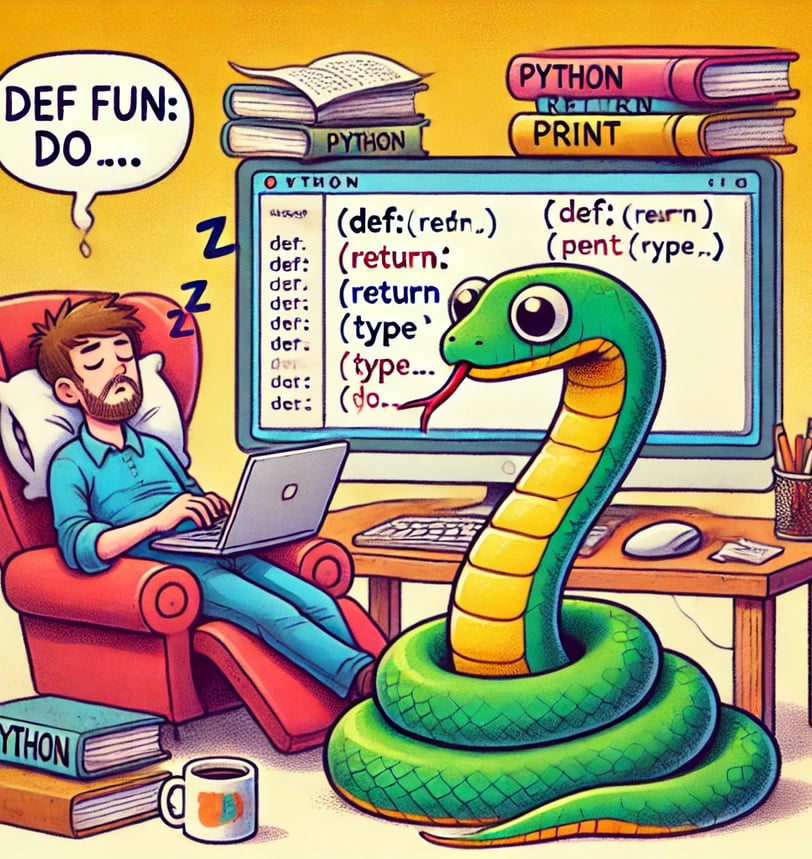
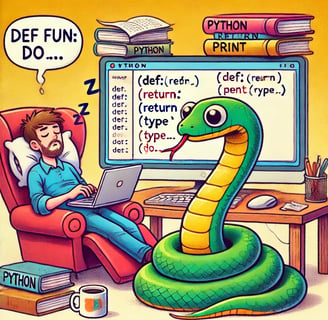
๐ What is a Function?
A function in Python is like a magic spell. You say its name, wave some parentheses, and voilร โit performs a task! Functions allow you to break down complex problems into smaller, manageable chunks. They help you write DRY (Don't Repeat Yourself) code, meaning you write something once and reuse it as often as you like.
โจ Why Use Functions?
Functions are the backbone of any organized Python program. Hereโs why you should use them:
Reusability: Write code once, use it a bazillion times.
Modularity: Make your code neat and organized, like a tidy sock drawer.
Readability: Break down code into readable chunks, so your future self doesn't hate you.
Maintainability: Fix bugs in one place, and the whole program benefits.
๐ How to Define and Call a Function
To define a function, use the def keyword, followed by the function name, parentheses (), and a colon :. Inside the function, write the magic spells (the code) you want to run.
๐ The Mighty return Statement
The return statement is like the grand finale of a magic trick. It gives back a result from the function to whoever called it. Without a return, the function performs its tricks and leaves without a word.
๐ฏ Parameters and Arguments
Parameters are like placeholders in your function definition. When you call the function, you fill these placeholders with arguments (the actual values).
๐ Keyword Arguments and Default Parameters
Keyword arguments are like ordering a customized pizzaโjust tell Python exactly what you want. Default parameters provide default values if no arguments are given.
๐ args and *kwargs: The Superstars of Flexibility
Sometimes, you want your function to accept an unknown number of arguments. Enter args and *kwargs.
*args: Allows a function to accept any number of positional arguments.
**kwargs: Allows a function to accept any number of keyword arguments.
๐งโโ๏ธ The Magic of Functions with a Docstring
A docstring is like a magic scroll attached to your function. It explains what your function does, so others (or future you) can understand its purpose.
๐ Using type() and dir() with Functions
โ๏ธ Deleting a Variable or Object with del
๐งฉ Fun Exercises - Master Your Function Skills!
Cube Function:
Greeting Function:
Temperature Conversion Program (temperature.py):
Flexible Pizza Order Function:
Hero Stats Function with Keyword Arguments:
๐ Kahoot Fun Awaits! ๐
To fully enjoy the Kahoot game, make sure you complete the loops section first. Itโs essential for understanding the functions and loops game. Get ready for some interactive learning and fun!
Happy playing! ๐ฎ