๐ 101-Unlock the Power of Python Dictionaries! ๐
PYTHON
8/31/20242 min read
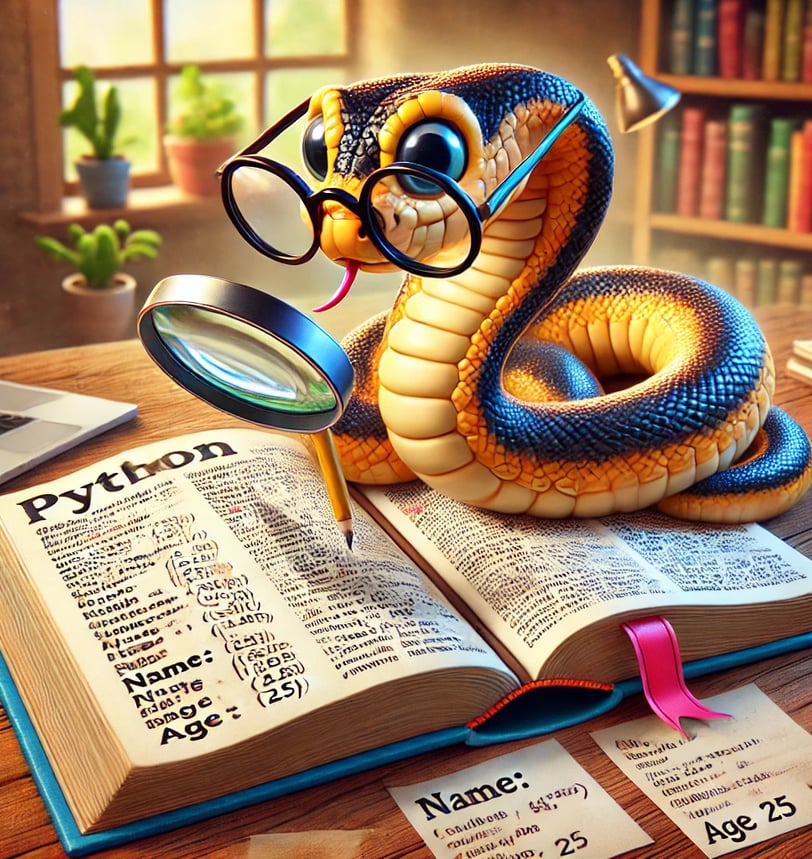
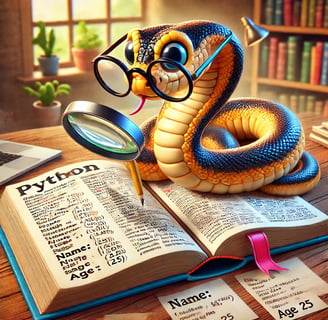
Welcome, Python enthusiasts! Today, we are diving deep into one of the most versatile and essential data structures in Python: Dictionaries. They are your go-to for storing data in key-value pairs, and they come with a wealth of methods and functionalities to make your coding life easier. So, letโs explore dictionaries step-by-step, with fun examples to illustrate their power! ๐
What is a Dictionary in Python? ๐
A dictionary in Python is an unordered, mutable, and indexed collection. This means:
Unordered: The items have no defined order.
Mutable: We can change, add, or remove items after the dictionary has been created.
Indexed: We can access dictionary items using a key.
Dictionaries store data in key-value pairs. Think of a real-world dictionary where you have a word (key) and its meaning (value). In Python, it looks like this:
Creating a Dictionary ๐ ๏ธ
You can create dictionaries in two main ways: using curly braces {} or the dict() function.
Method 1: Using Curly Braces
Method 2: Using the dict() Function
Both methods create a dictionary, but the dict() function is a bit more readable and allows you to omit the quotes around the keys.
Accessing Values ๐
To access values in a dictionary, you simply use the key inside square brackets [] or the get() method.
Note: Using my_dict[key] will raise a KeyError if the key is not found, while my_dict.get(key) will return None.
Adding and Modifying Elements โ
Dictionaries are mutable, so you can add new key-value pairs or modify existing ones:
Removing Elements โ
You can remove elements from a dictionary using the del keyword, pop(), or popitem() methods:
Dictionary Methods ๐ ๏ธ
Python provides several built-in methods to work with dictionaries:
dict.keys(): Returns a view object displaying a list of all the keys in the dictionary.
dict.values(): Returns a view object displaying a list of all the values.
dict.items(): Returns a view object displaying a list of all key-value pairs.
dict.update(): Updates the dictionary with elements from another dictionary or an iterable of key-value pairs.
dict.clear(): Removes all elements from the dictionary.
dict.copy(): Returns a shallow copy of the dictionary.
Example of Using Dictionary Methods
Looping Through Dictionaries ๐
You can loop through a dictionary to access its keys, values, or key-value pairs:
Checking Membership ๐
To check if a key exists in a dictionary, use the in keyword:
Real-World Use Cases ๐
Dictionaries are incredibly versatile and can be used in various scenarios:
1. Counting Frequencies of Elements ๐งฎ
Dictionaries are great for counting the frequency of elements in a collection. For example, counting the number of times each word appears in a text:
2. Storing Configuration Settings ๐ ๏ธ
Dictionaries are often used to store configuration settings for an application:
3. Representing Database Records ๐๏ธ
Dictionaries are perfect for representing records or data entries:
4. Caching or Memoization ๐
Dictionaries can store computed results for quick lookups, which is useful in caching or memoization:
Summary ๐
Dictionaries are mutable collections that store data in key-value pairs.
You can access, add, modify, and remove elements dynamically.
Python provides several built-in methods to manipulate dictionaries efficiently.
Ready to Test Your Knowledge? ๐ฏ
Take our Kahoot quiz to see how well you understand Python dictionaries!